Lisk Service
Lisk Service is a web application that allows interaction with various blockchain networks based on Lisk and Bitcoin protocols.
The main focus of Lisk Service is to provide data to the UI clients such as Lisk Desktop and Lisk Mobile. Lisk Service makes it possible to access all blockchain live data in a similar way to the regular Lisk SDK API, and in addition provides users with much more details and endpoints, such as geolocation and various statistics about network usage.
The project implementation is based on Microservices. The technical stack is designed to deliver several microservices, and each of them provides one particular functionality. The data is served in JSON format and exposed by a public RESTful API.
Architecture
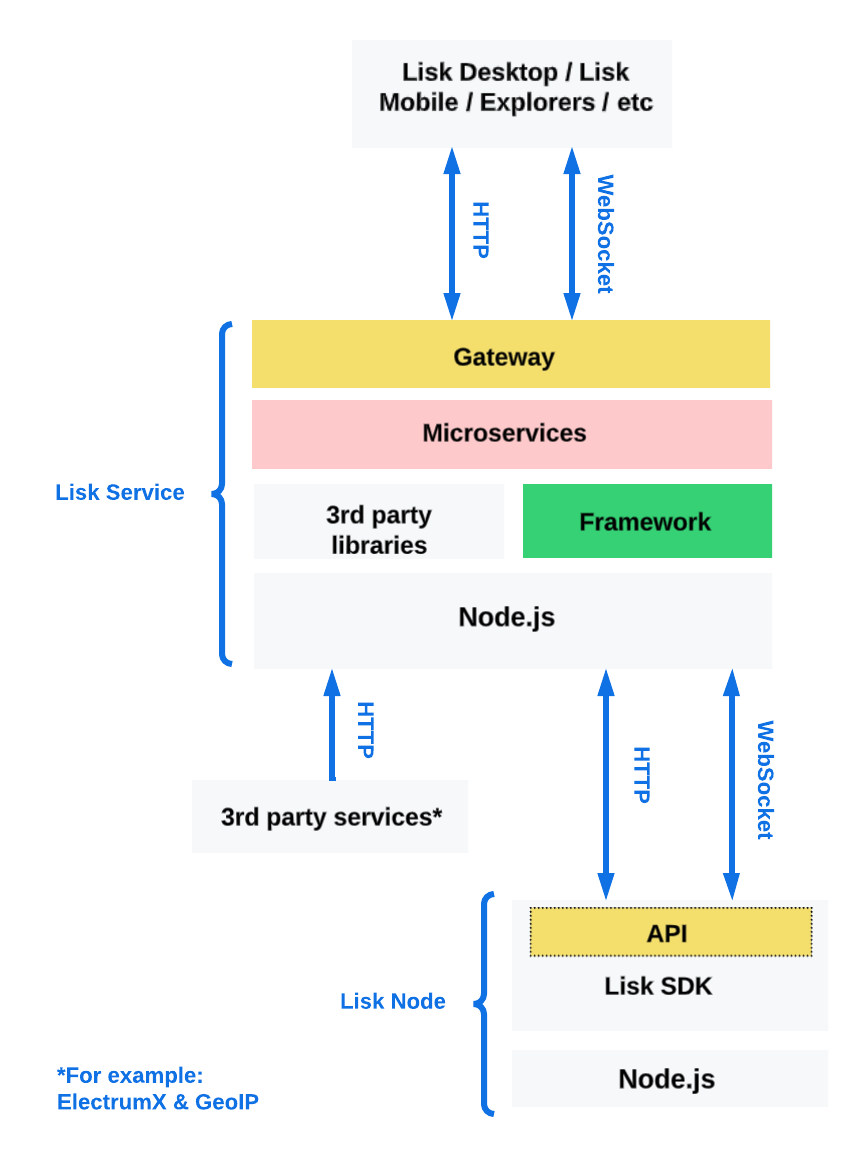
Microservices
Microservice | Description |
---|---|
Gateway |
The Gateway component provides a RESTful HTTP API, which all users of Lisk Service can access and use. Its main purpose is to proxy API requests from users to other components provided by Lisk Service. It also maintains backwards compatibility when its public API is changed or replaced by a new version. This provides users with a central point of data access that never breaks existing application compatibility. |
Lisk |
The REST client component acts as a bridge between the Lisk SDK and the Lisk Service Gateway. Its main purpose is to provide enriched data from the Lisk SDK API. This component is designed to provide high availability, together with efficient and reliable access to the Lisk SDK API. |
Market |
The Market service allows price data retrieval. It supports multiple sources to maintain the current up-to-date Lisk token price, and ensures this is available to the clients in real time. |
Newsfeed |
The Newsfeed service is a single-purpose microservice that aggregates content sharing platforms and shares collected data with UI clients such as Lisk Desktop. |
Template |
The Template service is an abstract service whereby all of the services from Lisk Service are inherited from. It allows all services to share a similar interface and design pattern. The purpose here is to reduce code duplication and increase consistency between each service, hence simplifying code maintenance and testing. |
APIs & Usage
Once Lisk Service is set up, configured and started, it is possible to retrieve data from the blockchain network.
Lisk Service provides the data through several alternative APIs:
The HTTP API
The HTTP API offers a RESTful API with various additional endpoints as compared to the HTTP API of a normal Lisk node.
This API can be utilized to build powerful wallets and user interfaces for blockchain applications which are built with the Lisk SDK.
Public Lisk Service APIs
There is a public HTTP API for every public Lisk blockchain network, which can be used to query the desired information from the network.
- Lisk Mainnet
-
-
Public API:
https://service.lisk.com/api/v2/
-
API specification: Lisk Service HTTP API reference (Mainnet)
-
- Lisk Testnet
-
-
Public API:
https://testnet-service.lisk.com/api/v2/
-
API specification: Lisk Service HTTP API reference (Testnet)
-
Example: Request data with curl
curl -X GET "http://localhost:9901/api/v2/forgers" -H "accept: application/json"
{
"data": [
{
"username": "spaceone_pool",
"totalVotesReceived": "24200000000000",
"address": "lsk52ox9f8t7oghqtbtytvpkqzv4wf2srjsvoaff7",
"minActiveHeight": 16357328,
"isConsensusParticipant": true,
"nextForgingTime": 1635159800
},
{
"username": "btf",
"totalVotesReceived": "17250000000000",
"address": "lsk49cfgah7bz84ncoqkhsvg5o2bsmj23x3vqo9y5",
"minActiveHeight": 16486490,
"isConsensusParticipant": true,
"nextForgingTime": 1635159810
},
// ...
{
"username": "gregoryh",
"totalVotesReceived": "21998000000000",
"address": "lskfkvo54opxs7cn6aefjhuupbr6zv7yegyow9sb4",
"minActiveHeight": 16467538,
"isConsensusParticipant": true,
"nextForgingTime": 1635160820
}
],
"meta": {
"count": 103,
"offset": 0,
"total": 103
}
}
The JSON-RPC API
The JSON-RPC API provides blockchain data in standardized JSON format over a WebSocket connection.
The API uses the socket.io
library and it is compatible with JSON-RPC 2.0 standard.
Check out the RPC endpoints (Lisk Service) reference for an overview of all available RPC requests.
- Lisk Mainnet
-
-
Public API:
wss://service.lisk.com/rpc-v2
-
- Lisk Testnet
-
-
Public API:
wss://testnet-service.lisk.com/rpc-v2
-
Example: Emit to remote-procedure calls with socket.io
node --version
# v16.15.0
npm i socket.io-client (1)
npm i jsome (2)
1 | Use the socket.io-client to connect to the RPC API. |
2 | Optionally install jsome to prettify the API response. |
// 1. Require the dependencies
const io = require('socket.io-client'); // The socket.io client
const jsome = require('jsome'); // Prettifies the JSON output
jsome.params.colored = true;
// Use local Service node
const WS_RPC_ENDPOINT = 'ws://localhost:9901/rpc-v2';
//Use public Service node
//const WS_RPC_ENDPOINT = "wss://service.lisk.com/rpc-v2";
// 2. Connect to Lisk Service via WebSockets
const socket = io(WS_RPC_ENDPOINT, {
forceNew: true,
transports: ['websocket']
});
// 3. Emit the remote procedure call
socket.emit('request', {
jsonrpc: '2.0',
method: 'get.forgers',
params: {limit: "5", offset: "0"} },
answer => {
// console.log(answer);
jsome(answer);
process.exit(0);
});
Run the above script with Node.js to receive the API response in the terminal:
node rpc.js
The Subscribe API
The Subscribe API, or sometimes called the Publish/Subscribe or Event-Driven API uses a two-way streaming connection, which means that not only the client can request the server for a data update, but also the server can notify the client about new data instantly as it arrives.
Check out the Publish/Subscribe API (Lisk Service) reference for an overview of all available RPC requests. |
- Lisk Mainnet
-
-
Public API:
wss://testnet-service.lisk.com/blockchain
-
- Lisk Testnet
-
-
Public API:
wss://testnet-service.lisk.com/blockchain
-
Example: Subscribe to events with socket.io
Use the socket.io-client to connect to the RPC API.
npm i socket.io-client
const io = require('socket.io-client');
const jsome = require('jsome');
jsome.params.colored = true;
// Uses local Service node
const WS_SUBSCRIBE_ENDPOINT = 'ws://localhost:9901/blockchain';
// Uses public Service node
//const WS_SUBSCRIBE_ENDPOINT = "wss://service.lisk.com/blockchain";
const socket = io(WS_SUBSCRIBE_ENDPOINT, {
forceNew: true,
transports: ['websocket'],
});
const subscribe = event => {
socket.on(event, answer => {
console.log(`====== ${event} ======`);
// console.log(answer);
jsome(answer);
});
};
subscribe('update.block');
subscribe('update.round');
subscribe('update.forgers');
subscribe('update.transactions.confirmed');
subscribe('update.fee_estimates');
// To log all events
[
'connect', 'reconnect',
'connect_error', 'connect_timeout', 'error', 'disconnect',
'reconnect', 'reconnect_attempt',
'reconnecting', 'reconnect_error', 'reconnect_failed',
].forEach(item => {
socket.on(item, res => {
console.log(`Event: ${item}, res: ${res || '-'}`);
});
});
// To log incoming data
['status'].forEach(eventName => {
socket.on(eventName, newData => {
console.log(
`Received data from ${WS_SUBSCRIBE_ENDPOINT}/${eventName}: ${newData}`,
);
});
});
Run the above script with Node.js to receive all published events from the Subscribe API:
node subscribe.js