Developer changelog
Updated type numbers for the default transaction types
SDK 2.3.7 Types |
SDK 3.0.2 Types |
Name |
---|---|---|
0 |
8 |
transfer |
1 |
9 |
second passphrase |
2 |
10 |
register delegate |
3 |
11 |
cast votes |
4 |
12 |
register multisignature |
The networkIdentifier parameter
The new parameter networkIdentifier
is introduced with Lisk SDK version 3.x.
It is now required to provide the networkIdentifier
of the specific network when creating a transaction.
This eliminates the possibility of transaction replays on different networks.
The networkIdentifier is required for creating a transaction object.
|
The network identifier is a hash value based on the following parameters:
-
The payload hash of the genesis block. (Example: Devnet genesis block payloadHash).
-
The Community identifier specified in the genesis block. (Example: Devnet genesis block Community Identifier).
const HelloTransaction = require('../hello_transaction');
const { EPOCH_TIME } = require('@liskhq/lisk-constants');
const {getNetworkIdentifier} = require('@liskhq/lisk-cryptography');
const networkIdentifier = getNetworkIdentifier(
"23ce0366ef0a14a91e5fd4b1591fc880ffbef9d988ff8bebf8f3666b0c09597d",
"Lisk",
);
const getTimestamp = () => {
// check config file or curl localhost:4000/api/node/constants to verify your epoc time
const millisSinceEpoc = Date.now() - Date.parse(EPOCH_TIME);
const inSeconds = ((millisSinceEpoc) / 1000).toFixed(0);
return parseInt(inSeconds);
};
const tx = new HelloTransaction({
asset: {
hello: 'world',
},
networkIdentifier: networkIdentifier,
timestamp: getTimestamp(),
});
tx.sign('creek own stem final gate scrub live shallow stage host concert they');
console.log(tx.stringify());
process.exit(0);
Updated genesis block for devnet
Due to the changes that have been introduced for the structure of transaction objects, the genesis block and it’s transactions were created new for Lisk SDK version 3.0.
See the new genesis block on Github: https://github.com/LiskHQ/lisk-sdk/blob/v3.0.2/sdk/src/samples/genesis_block_devnet.json
See the new devnet config: https://github.com/LiskHQ/lisk-sdk/blob/v3.0.2/sdk/src/samples/config_devnet.json
New genesis account
Name | Address | Passphrase | Description |
---|---|---|---|
Genesis account |
11237980039345381032L |
|
The genesis account holds all the tokens that are created in the devnet genesis block. It is utilized to vote for the forging genesis delegates in a convenient way during development. |
Database changes
To see what has changed in the database layer of Lisk SDK 3.x, compare the 2 figures Database layer SDK 2.x
and Database layer SDK 3.x
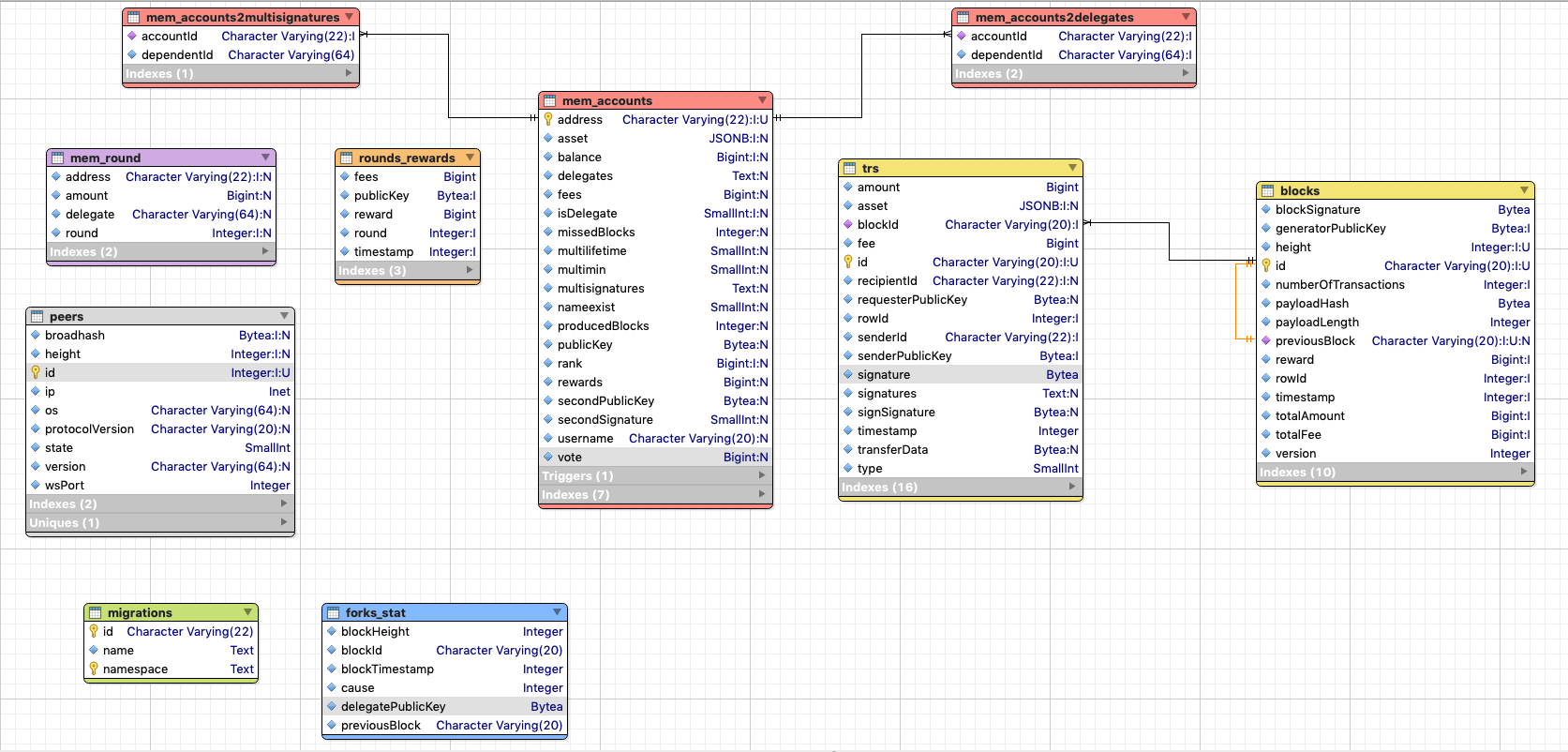
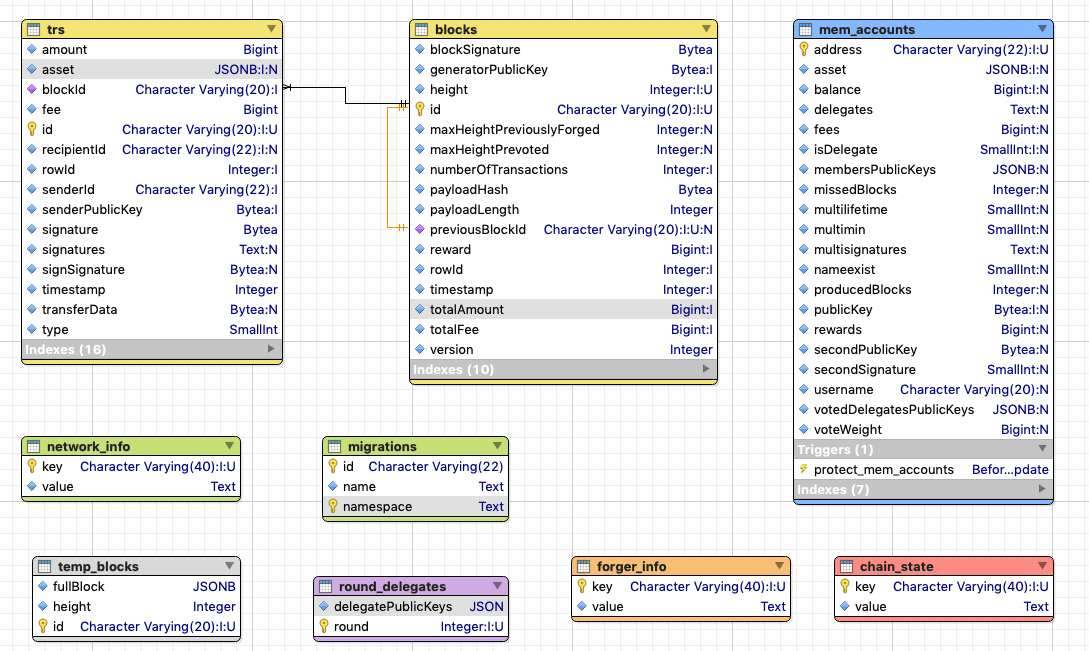
API changes
The list of API changes only comprises of the parts of the API that changed from Lisk SDK 2.x to 3.x. To see the complete API specification for Lisk SDK 3.x, please refer to the Config reference. |
Changes are grouped by the different API endpoints for the Lisk SDK.
Peers
Query
GET /peers
Parameters
Name | Description | Schema | Default | ||||||
---|---|---|---|---|---|---|---|---|---|
broadhash |
Broadhash of the network |
string (hex) |
|||||||
height |
Current height of the network |
integer (int32) |
|||||||
httpPort |
HTTP port of the node or delegate |
integer (int32) |
|||||||
ip |
IP of the node or delegate |
string (ip) |
|||||||
limit |
Limit applied to results |
integer (int32) |
|
||||||
offset |
Offset value for results |
integer (int32) |
|
||||||
os |
OS of the node |
string |
|||||||
protocolVersion |
Protocol version of the node |
string (protocolVersion) |
|||||||
sort |
Fields to sort results by |
enum (height:asc, height:desc, version:asc, version:desc) |
|
||||||
state |
Current state of the network |
|
|||||||
version |
Lisk version of the node |
string (version) |
|||||||
wsPort |
Web socket port for the node or delegate |
integer (int32) |
Peer object
Name | Description | Schema | ||||||
---|---|---|---|---|---|---|---|---|
height |
Network height on the peer node.
Represents the current number of blocks in the chain on the peer node. |
integer |
||||||
broadhash |
Broadhash on the peer node.
Broadhash is established as an aggregated rolling hash of the past five blocks present in the database. |
string (hex) |
||||||
nonce |
Unique Identifier for the peer. Random string. |
string (minLenght: 1) |
||||||
optional |
The port the peer node uses for HTTP requests, e.g. API calls. |
integer (int32) |
||||||
ip |
IPv4 address of the peer node. |
string (ip) |
||||||
networkId |
The network identifier as per LIP-0009 |
string |
||||||
os |
The Operating system, that the peer node runs on. |
string |
||||||
protocolVersion |
The protocol version of Lisk Core that the peer node runs on. |
string (protocolVersion) |
||||||
state |
The state of the Peer. |
|
||||||
version |
The version of Lisk Core that the peer node runs on. |
string (version) |
||||||
wsPort |
The port the peer node uses for websocket connections, e.g. P2P broadcasts. |
integer (int32) |
Blocks
Block object
Name | Description | Schema |
---|---|---|
blockSignature |
Derived from a SHA-256 hash of the block header,
that is signed by the private key of the delegate who forged the block. |
string (signature) |
confirmations |
Number of times that this Block has been confirmed by the network.
By forging a new block on a chain, all former blocks in the chain get confirmed by the forging delegate. |
integer |
generatorAddress |
Lisk address of the delegate who forged the block. |
string (address) |
generatorPublicKey |
Public key of the delegate who forged the block. |
string (publicKey) |
height |
Height of the network, when the block was forged.
The height of the networks represents the number of blocks,
that have been forged on the network since the Genesis block. |
integer |
id |
Unique identifier of the block.
Derived from the block signature. |
string (id) |
maxHeightPreviouslyForged |
Largest height of any block previously forged by the generatorPublicKey as defined in the Lisk BFT Protocol.
See https://github.com/LiskHQ/lips/blob/master/proposals/lip-0014.md |
integer |
maxHeightPrevoted |
Largest height of an ancestor block with at least 68 prevotes as defined in the Lisk BFT Protocol.
See https://github.com/LiskHQ/lips/blob/master/proposals/lip-0014.md |
integer |
numberOfTransactions |
The number of transactions processed in the block. |
integer |
payloadHash |
Hash of the payload of the block.
The payload of a block is comprised of the transactions the block contains.
For each type of transaction exists a different maximum size for the payload. |
string (hex) |
payloadLength |
Bytesize of the payload hash. |
integer |
previousBlockId |
The id of the previous block of the chain. |
string (id) |
reward |
The Lisk reward for the delegate. |
string |
timestamp |
Unix timestamp |
integer |
totalAmount |
The total amount of Lisk transferred. |
string |
totalFee |
The total amount of fees associated with the block. |
string |
totalForged |
Total amount of LSK that have been forged in this Block.
Consists of fees and the reward. |
string |
version |
Versioning for future upgrades of the lisk protocol. |
integer |
Delegates
Query
GET /delegates
Parameters
Type | Name | Description | Schema | Default | |||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Query |
address |
Address of an account |
string (address) |
||||||||||||||||
Query |
limit |
Limit applied to results |
integer (int32) |
|
|||||||||||||||
Query |
offset |
Offset value for results |
integer (int32) |
|
|||||||||||||||
Query |
publicKey |
Public key to query |
string (publicKey) |
||||||||||||||||
Query |
search |
Fuzzy delegate username to query |
string |
||||||||||||||||
Query |
secondPublicKey |
Second public key to query |
string (publicKey) |
||||||||||||||||
Query |
sort |
Fields to sort results by |
|
|
|||||||||||||||
Query |
username |
Delegate username to query |
string (username) |
Delegate
Name | Description | Schema |
---|---|---|
approval |
Percentage of the voters weight, that the delegate owns in relation to the total supply of Lisk. |
number |
missedBlocks |
Total number of blocks the delegate has missed. |
integer |
producedBlocks |
Total number of blocks the delegate has forged. |
integer |
productivity |
Productivity rate.
Percentage of successfully forged blocks (not missed) by the delegate. |
number |
rewards |
Total sum of block rewards that the delegate has forged. |
string |
username |
The delegates' username.
A delegate chooses the username by registering a delegate on the Lisk network.
It is unique and cannot be changed later. |
string (username) |
voteWeight |
The voters weight of the delegate.
Represents the total amount of Lisk (in Beddows) that the delegates' voters own.
The voters weight decides which rank the delegate gets in relation to the other delegates and their voters weights. |
string |
vote |
The voters weight of the delegate.
Represents the total amount of Lisk (in Beddows) that the delegates' voters own.
The voters weight decides which rank the delegate gets in relation to the other delegates and their voters weights. |
string |
rank |
The voters weight of the delegate.
Rank of the delegate.
The rank is defined by the voters weight/ approval of a delegates, in relation to all other delegates. |
integerOrNull |
DelegateWithAccount
Name | Description | Schema |
---|---|---|
account |
||
approval |
Percentage of the voters weight, that the delegate owns in relation to the total supply of Lisk. |
number |
missedBlocks |
Total number of blocks the delegate has missed. |
integer |
producedBlocks |
Total number of blocks the delegate has forged. |
integer |
productivity |
Productivity rate.
Percentage of successfully forged blocks (not missed) by the delegate. |
number |
rewards |
Total sum of block rewards that the delegate has forged. |
string |
username |
The delegates' username.
A delegate chooses the username by registering a delegate on the Lisk network.
It is unique and cannot be changed later. |
string (username) |
voteWeight |
The voters weight of the delegate.
Represents the total amount of Lisk (in Beddows) that the delegates' voters own.
The voters weight decides which rank the delegate gets in relation to the other delegates and their voters weights. |
string |
vote |
The voters weight of the delegate.
Represents the total amount of Lisk (in Beddows) that the delegates' voters own.
The voters weight decides which rank the delegate gets in relation to the other delegates and their voters weights. |
string |
rank |
The voters weight of the delegate.
Rank of the delegate.
The rank is defined by the voters weight/ approval of a delegates, in relation to all other delegates. |
integerOrNull |
Node
Query node/transactions/state
GET /node/transactions/{state}
Parameters
Type | Name | Description | Schema | Default |
---|---|---|---|---|
Path |
state |
State of transactions to query |
enum (pending, ready, received, validated, verified) |
|
Query |
id |
Transaction id to query |
string (id) |
|
Query |
limit |
Limit applied to results |
integer (int32) |
|
Query |
offset |
Offset value for results |
integer (int32) |
|
Query |
recipientId |
Recipient’s Lisk address |
string (address) |
|
Query |
recipientPublicKey |
Recipient’s public key |
string (publicKey) |
|
Query |
senderId |
Sender’s Lisk address |
string (address) |
|
Query |
senderPublicKey |
Sender’s public key |
string (publicKey) |
|
Query |
sort |
Fields to sort results by |
enum (amount:asc, amount:desc, fee:asc, fee:desc, type:asc, type:desc, timestamp:asc, timestamp:desc) |
|
Query |
type |
Transaction type (0-*) |
integer |
NodeConstants object
Name | Description | Schema |
---|---|---|
build |
The build number.
Consists of |
string |
commit |
The last commit that was added to the codebase. |
string |
epoch |
Timestamp of first block on the network. |
string (date-time) |
fees |
||
milestone |
The Reward, each forger will get for forging a block at the current slot.
After a certain amount of slots, the reward will be reduced. |
string |
nethash |
Describes the network. The nethash describes e.g. the Mainnet or the Testnet, that the node is connecting to. |
string |
nonce |
Unique identifier of the node. Random string. |
string |
networkId |
Unique identifier for the network.
The networkId that the node is connecting to, see LIP-0009 for more details. |
string |
protocolVersion |
The Lisk Core protocol version, that the node is running on. |
string (protocolVersion) |
reward |
The reward a delegate will get for forging a block.
Dependant on the slot height. |
string |
supply |
Total supply of LSK in the network. |
string |
version |
The Lisk Core version, that the node is running on. |
string (version) |
NodeStatus object
Name | Description | Schema |
---|---|---|
chainMaxHeightFinalized |
The largest height with precommits by at least 68 delegates.
See https://github.com/LiskHQ/lips/blob/master/proposals/lip-0014.md |
integer |
broadhash |
Broadhash is established as an aggregated rolling hash of the past five blocks present in the database.
Broadhash consensus serves a vital function for the Lisk network in order to prevent forks.
It ensures that a majority of available peers agree that it is acceptable to forge. |
string |
consensus |
Percentage of the connected peers, that have the same broadhash as the querying node. |
integer |
loaded |
True if the blockchain loaded. |
boolean |
networkHeight |
Current block height of the network.
Represents the current number of blocks in the chain on the network. |
integer |
transactions |
Transactions known to the node. |
object |
currentTime |
Current time of the node in miliseconds, (Unix timestamp). |
integer |
height |
Current block height of the node.
Represents the current number of blocks in the chain on the node. |
integer |
secondsSinceEpoch |
Number of seconds that have elapsed since the Lisk epoch time, (Lisk timestamp). |
integer |
syncing |
True if the node syncing with other peers. |
boolean |
Transactions
Query
GET /transactions
Parameters
Type | Name | Description | Schema | Default |
---|---|---|---|---|
Query |
blockId |
Block id to query |
string (id) |
|
Query |
data |
Fuzzy additional data field to query |
string (additionalData) |
|
Query |
fromTimestamp |
Starting unix timestamp |
integer |
|
Query |
height |
Current height of the network |
integer (int32) |
|
Query |
id |
Transaction id to query |
string (id) |
|
Query |
limit |
Limit applied to results |
integer (int32) |
|
Query |
maxAmount |
Maximum transaction amount in Beddows |
integer |
|
Query |
minAmount |
Minimum transaction amount in Beddows |
integer |
|
Query |
offset |
Offset value for results |
integer (int32) |
|
Query |
recipientId |
Recipient’s Lisk address |
string (address) |
|
Query |
recipientPublicKey |
Recipient’s public key |
string (publicKey) |
|
Query |
senderId |
Sender’s Lisk address |
string (address) |
|
Query |
senderIdOrRecipientId |
Lisk address |
string (address) |
|
Query |
senderPublicKey |
Sender’s public key |
string (publicKey) |
|
Query |
sort |
Fields to sort results by |
enum (amount:asc, amount:desc, fee:asc, fee:desc, type:asc, type:desc, timestamp:asc, timestamp:desc) |
|
Query |
toTimestamp |
Ending unix timestamp |
integer |
|
Query |
type |
Transaction type (0-*) |
integer |
Transactionrequest
Name | Description | Schema |
---|---|---|
asset |
Displays additional transaction data. For example, this can include the vote data or delegate username. |
|
amount |
Amount of Lisk to be transferred in this transaction. |
string |
fee |
Transaction fee associated with this transaction. |
string |
recipientId |
Lisk Address of the Recipients' account. |
string (address) |
id |
Unique identifier of the transaction.
Derived from the transaction signature. |
string (id) |
senderPublicKey |
The public key of the sender’s account. |
string (publicKey) |
signSignature |
Contains the second signature, if the transaction is sent from an account with second passphrase activated. |
string (signature) |
signature |
Derived from a SHA-256 hash of the transaction object,
that is signed by the private key of the account who created the transaction. |
string (signature) |
signatures |
If the transaction is a multisignature transaction, all signatures of the members of the corresponding multisignature group will be listed here. |
string (signature) array |
timestamp |
Time when the transaction was created.
Unix timestamp. |
integer |
type |
Describes the transaction type. |
integer |
Transaction asset
Name | Description | Schema |
---|---|---|
amount |
Amount of Lisk to be transferred in this transaction. |
string |
recipientId |
Lisk address of the Recipients' account. |
string (address) |
Transaction object
Name | Description | Schema | ||||
---|---|---|---|---|---|---|
asset |
object |
|||||
amount |
Amount of Lisk to be transferred in this transaction. |
string |
||||
recipientId |
Lisk Address of the Recipients' account. |
string (address) |
||||
blockId |
The Id of the block, this transaction is included in. |
string (id) |
||||
confirmations |
Number of times that this transaction has been confirmed by the network.
By forging a new block on a chain, all former blocks and their contained transactions in the chain get confirmed by the forging delegate. |
integer |
||||
fee |
Transaction fee associated with this transaction. |
string |
||||
height |
The height of the network, at the moment where this transaction was included in the blockchain. |
integer |
||||
id |
Unique identifier of the transaction.
Derived from the transaction signature. |
string (id) |
||||
ready |
Only present in transactions sent from a multisignature account, or transactions type 4 (multisignature registration).
False, if the minimum amount of signatures to sign this transaction has not been reached yet.
True, if the minimum amount of signatures has been reached. |
boolean |
||||
receivedAt |
The timestamp of the moment, where a node discovered a transaction for the first time. |
string (date-time) |
||||
senderId |
Lisk Address of the Senders' account. |
string (address) |
||||
senderPublicKey |
The public key of the sender’s account. |
string (publicKey) |
||||
recipientPublicKey |
The public key of the recipient’s account. |
string (publicKey) |
||||
senderSecondPublicKey |
The second public key of the sender’s account, if it exists. |
string (publicKey) |
||||
signSignature |
Contains the second signature, if the transaction is sent from an account with second passphrase activated. |
string (signature) |
||||
signature |
Derived from a SHA-256 hash of the transaction object,
that is signed by the private key of the account who created the transaction. |
string (signature) |
||||
signatures |
string (signature) array |
|||||
timestamp |
Time when the transaction was created.
Unix timestamp. |
integer |
||||
type |
Describes the transaction type.
Minimum value : |
integer |